3. Conditionals#
In this notebook, we cover the following subjects:
Boolean Values and Expressions;
Conditional Statements;
User-Input.
3.1. Boolean Values and Expressions#
Stemming from Boolean Logic, let’s talk about another data type: Boolean values. This data type can only have two values, namely True or False, and is often used to represent the outcome of a condition or expression.
is_sunny: bool = True
is_raining: bool = False
A boolean expression is an expression that evaluates to True
or False
.
Note
The typehint for a variable that stores a boolean value is bool
(see example code above).
num_x: int = 1
num_y: int = 5
result: bool = num_x > num_y
print(result)
False
Note
The above code will print False since the variable y
stores the value of 5, which is greater then the value 1 stored in variable x
. In simple terms, 1 > 5 is False.
3.1.1. Comparison Operators#
To perform comparisons between values or expressions, we use what are called comparison operators. While these operations might already be familiar to you, the symbols used in Python are different from the standard mathematical symbols.
Operator |
Purpose |
---|---|
|
x is equal to y |
|
x is not equal to y |
|
x is greater than y |
|
x is less than y |
|
x is greater than or equal to y |
For example, using ==
we check whether the following expression is True
. You can think of the ==
operator as a way of checking if the value on the left side is precisely equal (same) to the value on the right side.
result: bool = (5 == 5)
print(result)
print('-------------------')
result2: bool = (1 == 3)
print(result2)
True
-------------------
False
Note
There is a clear difference between the use of =
and ==
: the former is an assignment operator, while the latter is a relational operator.
3.1.2. Logical Operators#
Now, with logical operators we can combine multiple boolean expressions and return a True
or False
value. In Python, there are three logical operators: and
, or
, and not
.
Operator |
Technical name |
Math symbol |
Python construct |
---|---|---|---|
And |
Conjunction |
\(\land\) |
|
Or |
Disjunction |
\(\lor\) |
|
Not |
Negation |
\(\lnot\) |
|
The
and
Operator: returnsTrue
if all expressions areTrue
.
A |
B |
A ∧ B |
---|---|---|
True |
True |
True |
True |
False |
False |
False |
True |
False |
False |
False |
False |
age: int = 17
is_adult: bool = (age >= 18) and (age < 65)
print(is_adult)
False
The
or
Operator: returnsTrue
if at least one expression holdsTrue
.
A |
B |
A ∨ B |
---|---|---|
True |
True |
True |
True |
False |
True |
False |
True |
True |
False |
False |
False |
day: str = "Saturday"
is_weekend: bool = (day == "Saturday") or (day == "Sunday")
print(is_weekend)
True
The
not
Operator: negates a boolean expression.
A |
¬A |
---|---|
True |
False |
False |
True |
B |
¬B |
---|---|
True |
False |
False |
True |
is_sunny: bool = True
not_sunny: bool = not is_sunny
print(not_sunny)
False
3.2. Conditional Statements#
Now that we’ve covered boolean values and expressions, let’s move on to a new concept: conditional statements. In order to write useful code, we want to be able to change the behavior of the program based on the fulfillment of certain conditions. Conditional statements allow us to do this, as Boolean expressions are used to determine which blocks of code to execute. The three types of conditional statements are if
, elif
, and else
.
1. if-statement: this is the simplest form of a conditional statement. If the condition after the if
holds True
, then the following statement is executed. The syntax is as follows:
if condition:
# Code block executes if condition is True
Note
Python is indentation-sensitive, meaning that indentation is used to show the hierarchy and structure of your code.
An example:
# What happens when we run this cell?
temperature: int = 5
if temperature < 0:
print("It's freezing.")
As you might notice, an if-statement is very useful when you want to run a specific piece of code only if a condition is true. However, this approach is limited because the code stops executing if the condition is not true, reducing flexibility. To account for this problem, we introduce the else-statement.
2. else-statement: if the condition is of the if-statement is False
, the body of the else
section will be executed. Together, we call this an if-else statement, and it has the following syntax:
if condition:
# Code block if condition is True
else:
# Code block if condition is False
temperature: int = 5
if temperature < 0:
print("It's freezing.")
else:
print("No frostbite today! Maybe go for a brisk walk?")
No frostbite today! Maybe go for a brisk walk?
You might be thinking that the additional else-statement still limits the response to only two possibilities. To overcome this issue, you can use the elif-statement and check for multiple conditions.
elif-statement:
elif
is an abbreviation of else if and allows you to check multiple conditions. If the preceeding conditions evaluate toFalse
, the firstelif
block that holdsTrue
gets executed. Note: in contrast to the else-statement, after theelif
keyword you should always define a new Boolean expression.
if condition1:
# Code block if condition1 is True
elif condition2:
# Code block if condition2 is True
else:
# Code block if all conditions are False
temperature: int = 5
if temperature < 0:
print("It's freezing! Time to build a snowman!")
elif 0 <= temperature < 15:
print("It's a bit chilly, perfect weather for a cozy sweater.")
else:
print("Nice weather! How about a picnic in the park?")
It's a bit chilly, perfect weather for a cozy sweater.
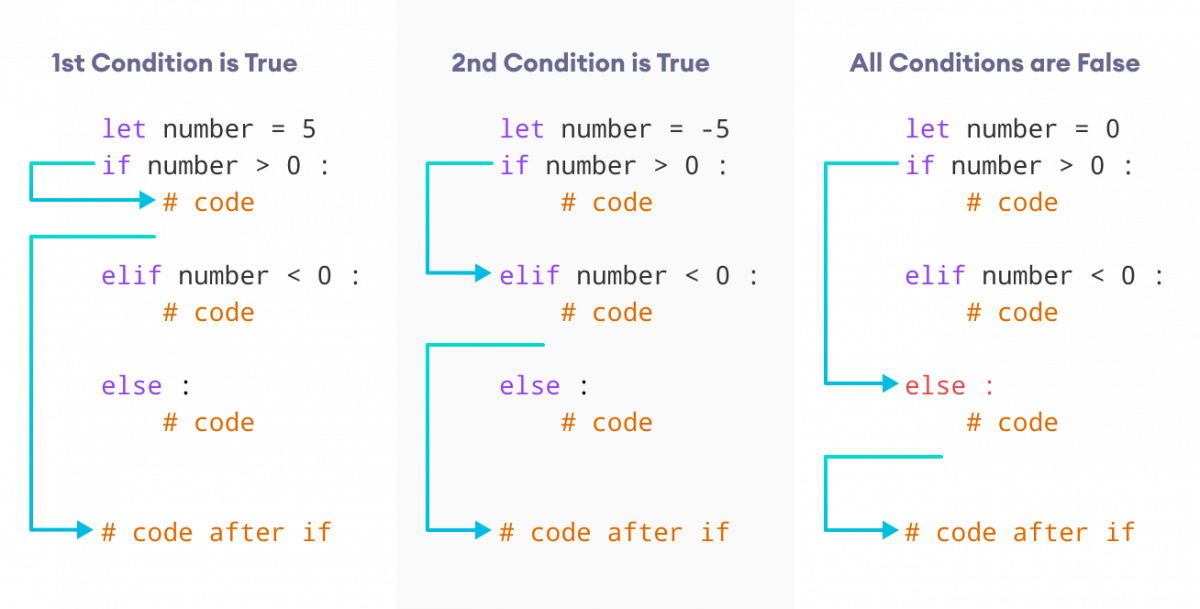
When there are more than two alternatives, therefore using if
, else
, and elif
, we refer to this as a chained conditional. As shown above, chained conditions are are checked from top to bottom, and if more than one condition evaluates to True
only the first branch for which the condition succeeds is executed.
Note
There is no limit to the amount of elif
blocks you wish to use, but remember to start with an if
statement. While an else
block at the end is optional, you can also end the statement with an elif
.
3.2.1. Importance of Indentation#
As you will see in the code snippet bellow, we used different levels of indentation. You have already used indentation in the previous chapter (Functions), but it is very important that you fully understand its meaning and power.
So what is indentation?
In Python, indentation isn’t just for making code look nice—it’s how the language knows what code belongs together. When you write a
loop
,function
, orconditional
(like anif statement
), everything that should happen inside it needs to be indented.If you forget or mix up spaces and tabs, Python will give you an error.
Here’s an example:
if x > 5:
print("x is greater than 5") # This line is indented, so it's part of the if-statement
print("This runs no matter what") # No indentation, so it's outside the if-statement
The indented part runs only if the condition is true, while the non-indented code runs regardless. So, Python uses indentation to tell what should happen inside or outside certain blocks of code.
Keep it neat and consistent, and you’ll be good!
Note
In Python, we usually use 4 spaces or 1 tab to indent.
3.2.2. Let’s think#
Let’s test our understanding! Answer the following questions regarding the piece of code underneath:
Question 1: Would the output change if I changed
elif
toif
?Question 2: What would happen if we set each character
trait = False
? (e.g.,brave = False
)Question 3: What would happen if we set only the
brave
variable to false:brave = False?
MINIMUM_AGE: int = 11
house: str = "?"
brave: bool = True
smart: bool = True
friendly: bool = True
age: int = 12
if age > MINIMUM_AGE:
if brave and friendly:
house = "Gryffindor"
elif smart:
house = "Slytherin"
print("You belong in {housename}!".format(housename = house))
else:
print("Wait till you're old enough to get your letter.")
You belong in Gryffindor!
And what will be the output of the following cell?
can_fly_a_Firebolt: bool = True
can_catch_a_Snitch: bool = True
can_hit_a_Bludger: bool = True
has_vertigo: bool = False
qualified: bool = not has_vertigo and can_fly_a_Firebolt and (can_catch_a_Snitch or can_hit_a_Bludger)
message: str = "Welcome to the team!" if qualified else "Better luck next time!"
print(message)
Welcome to the team!
3.2.3. Nested Conditionals#
One conditional can also be nested within another. That is, you can have a conditional statement within the body of one of the alternative executions of a conditional.
if condition1:
if condition2:
# Code block for both condition1 and condition2 being True
else:
# Code block for condition1 being True and condition2 being False
else:
# Code block for condition1 being False
day: str = "Saturday"
weather: str = "sunny"
if day == "Saturday" or day == "Sunday":
print("It's the weekend!")
if weather == "sunny":
print("Perfect day for a hike!")
else:
print("Maybe visit a museum or watch a movie.")
else:
print("It's a weekday.")
if weather == "rainy":
print("Good day to stay in and read a book.")
else:
print("How about a nice walk after work?")
It's the weekend!
Perfect day for a hike!
3.3. User input#
A program in Python can interact with the user using the input()
function. This function asks the user for input and returns the written answer as a string.
age = int(input("Enter your age: "))
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
---------------------------------------------------------------------------
StdinNotImplementedError Traceback (most recent call last)
Cell In[13], line 1
----> 1 age = int(input("Enter your age: "))
3 if age >= 18:
4 print("You are an adult.")
File /opt/hostedtoolcache/Python/3.8.18/x64/lib/python3.8/site-packages/ipykernel/kernelbase.py:1281, in Kernel.raw_input(self, prompt)
1279 if not self._allow_stdin:
1280 msg = "raw_input was called, but this frontend does not support input requests."
-> 1281 raise StdinNotImplementedError(msg)
1282 return self._input_request(
1283 str(prompt),
1284 self._parent_ident["shell"],
1285 self.get_parent("shell"),
1286 password=False,
1287 )
StdinNotImplementedError: raw_input was called, but this frontend does not support input requests.
Why do we use the int()
in the first line of the code-cell?
input() : This function prompts the user for input, and whatever the user types is returned as a string by default.
int(input()) : This converts the user’s input (which is a string) into an integer. It is used when you expect a number to be entered. If the user types something that can’t be converted to an integer, it will raise a ValueError
.
Answer : Therefore, we use int(input())
code, since we want the user to enter an integer. Moreover, we do not want to accept any other data type apart from integers.
3.4. Exercises#
Let’s practice! Mind that each exercise is designed with multiple levels to help you progressively build your skills. Level 1 is the foundational level, designed to be straightforward so that everyone can successfully complete it. In Level 2, we step it up a notch, expecting you to use more complex concepts or combine them in new ways. Finally, in Level 3, we get closest to exam level questions, but we may use some concepts that are not covered in this notebook. However, in programming, you often encounter situations where you’re unsure how to proceed. Fortunately, you can often solve these problems by starting to work on them and figuring things out as you go. Practicing this skill is extremely helpful, so we highly recommend completing these exercises.
For each of the exercises, make sure to add type hints
, and docstrings
(if applicable), moreover do not import any libraries unless specified otherwise.
3.4.1. Exercise 1#
Level 1: Using input()
, ask the user of your program to enter an integer number and checks whether it is a multiple of 6, 8, or both. You can assume that the input is a valid positive integer. The program should output a message using an f-string to indicate the result.
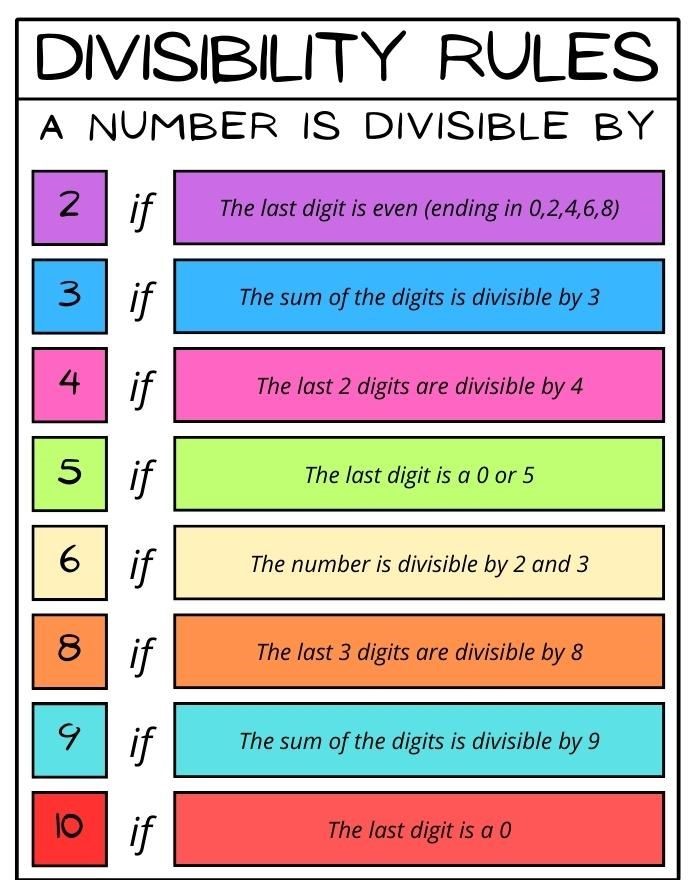
Example 1 input:
user_input: int = 24 # note that 24 is the obtained value from the user input
Example 1 output:
"The number 24 is a multiple of both 6 and 8."
Example 2 input:
user_input: int = 16 # note that 16 is the obtained value from the user input
Example 2 output:
"The number 16 is a multiple of 8 but not of 6."
# TODO.
Level 2: Write a script that asks the user to enter a number representing the current temperature in Celsius, then converts it to Fahrenheit. Based on the converted temperature, give a recommendation on whether the user should wear a jacket.
Follow these steps:
Convert the Celsius input to Fahrenheit using this formula: F = (C * 9/5) + 32.
If the Fahrenheit temperature is below 50°F, print:
It’s pretty cold! Definitely wear a jacket.
If it’s between 50°F and 68°F (inclusive), print:
It’s cool out. A light jacket is a good idea.
If it’s above 68°F, print:
It’s warm enough. No jacket needed!
Be sure to include exception handling to catch cases where the user enters something that’s not a number. If that happens, print: Invalid input. Please enter a numeric value for the temperature.
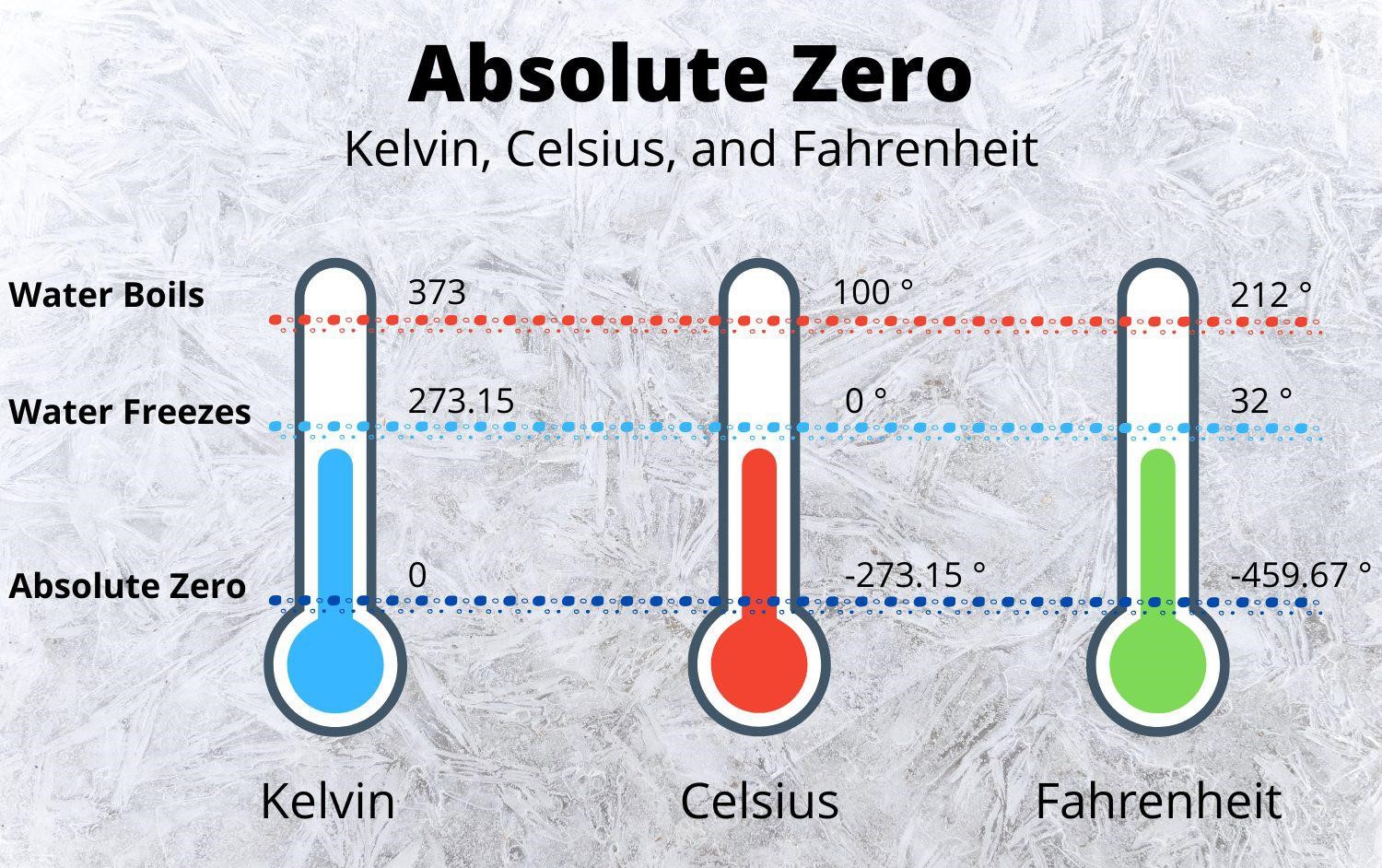
Example input:
Enter the temperature using Celsius: -5
Example output:
'It’s pretty cold! Definitely wear a jacket.'
# TODO.
Level 3: Write a function called is_prime_number()
that:
Prompts the user to input a number.
Checks if the number is prime.
If the number is prime, print “The number is prime!”.
If the number is not prime, print “The number is not prime!”.
Hint: A number is prime if it is greater than 1 and has no divisors other than 1 and itself.
Example input:
Enter a number: 29
Example output:
'The number is prime!'
# TODO.
3.4.2. Exercise 2#
Level 1: Write a program that prints “Hello World”
if both of the following conditions are met:
The variable
a
is greater than the variableb
.The variable
a
is an even number.
If either condition is not met, print “Bye World”
instead.
Example input:
a: int = 26
b: int = 20
Example output:
'Hello World'
# TODO.
Level 2: Imagine that ChatGPT can handle different types of conversation topics. Create a function chatgpt_topic_assist()
that takes three integer parameters:
seriousness (1-10): how serious the conversation is (1 is very casual, 10 is very serious).
length (in minutes): how long the conversation is expected to last).
randomness (1-10): how random the conversation is (1 is very structured, 10 is very unpredictable).
The function should classify ChatGPT’s “conversation type” based on these inputs:
“Deep philosophical debate” if seriousness >= 8 and length > 30 and randomness <= 3.
“Casual chit-chat” if seriousness <= 3 and length <= 15.
“Creative brainstorming” if randomness >= 7 and length > 20.
“Focused technical discussion” if seriousness >= 7 and randomness <= 4 and length <= 30.
Otherwise, return “General conversation”.
Add checks to ensure seriousness, length, and randomness fall within the appropriate ranges, and return “Invalid input” if they don’t.
Example input:
seriousness: int = 9
length: int = 45
randomness: int = 2
Example output:
'Deep philosophical debate'
# TODO.
Level 3: Create a Python function called guess_number()
that implements a number guessing game with a maximum of 3 attempts. The function should:
Generate a Random Number: Use the random module to generate a random integer between 1 and 10 (inclusive) - info about the random module here.
Allow Multiple Attempts: The user has a maximum of 3 guesses to find the correct number.
Ask for User Input: Prompt the user to guess the number.
Check the Guess: Compare the user’s guess to the generated number.
If the guess is correct, print a congratulatory message. (
‘Congratulations! You guessed the correct number.’
)If the guess is incorrect, print a message indicating whether the guess was too high or too low. (
’Too high/low! Try again.’
)
After the maximum number of attempts is reached, reveal the correct number and inform the user they have run out of attempts. (’Better luck next time, you guessed too high/low and ran out of attempts. The correct number was x.’
)
Example input:
Guess a number between 1 and 10: 3
Guess a number between 1 and 10: 8
Guess a number between 1 and 10: 7
Example output:
'Too low! Try again.'
'Too high! Try again.'
'Congratulations! You guessed the correct number.'
# TODO.
3.4.3. Exercise 3#
Level 1: Write a script that asks the user to rate a restaurant between 0 and 5. Depending on the rating, the program should give a specific recommendation. Here are the rating categories and the corresponding messages:
If the rating falls between 3.5 (inclusive) and 5 (inclusive), print:
Definitely worth your time! Give it a go.
If the rating is between 2.5 (inclusive) and 3.5 (exclusive), print:
It’s probably worth a shot.
If the rating is less than 2.5 (exclusive), print:
You might want to consider other options.
Ensure your program handles the ratings appropriately and uses f-strings for output if needed.
Hint:
Think about wether you should use int(input())
or just input()
. Which one would allow you to deal with both floats and integers?
Example input:
rating_restaurant: float = 4.6 # note that 4.6 is the obtained value from the user input
Example output:
'Definitely worth your time! Give it a go.'
# TODO.
Level 2: Write a function called basic_calculator()
that:
Prompts the user for two numbers.
Prompts the user to choose an operation: addition (+), subtraction (-), multiplication (*), or division (/).
Uses conditionals to determine which arithmetic operation to perform.
Performs the chosen arithmetic operation on the two numbers.
Returns the result of the operation.
If the user enters an invalid operation, it should print an error message.
Example input:
Enter the first number: 10
Enter the second number: 5
Choose an operation (+, -, *, /): *
Example output:
'The result is: 50'
# TODO.
Level 3: Write a function called custom_leap_year_validator()
that takes 1 parameter (year
) and checks whether the given year is a leap year based on the following custom rules:
A year is a leap year if:
It is divisible by 4 but not divisible by 100, or
It is divisible by 400, and
It is not divisible by 3200.
Additionally, for years divisible by 2000:
The year is not a leap year, even if it satisfies the above conditions.
Return True
if the year is a leap year, and False
otherwise.
Example inputs: you pass this in a function call.
custom_leap_year_validator(2024)
custom_leap_year_validator(2100)
custom_leap_year_validator(2000)
custom_leap_year_validator(2400)
custom_leap_year_validator(2404)
Example output:
True (leap year)
False (not a leap year)
False (because of the special rule for divisible by 2000)
False (divisible by 100)
True (leap year)
# TODO.
Material for the VU Amsterdam course “Introduction to Python Programming” for BSc Artificial Intelligence students. These notebooks are created using the following sources:
Learning Python by Doing: This book, developed by teachers of TU/e Eindhoven and VU Amsterdam, is the main source for the course materials. Code snippets or text explanations from the book may be used in the notebooks, sometimes with slight adjustments.