1. Variables, Expressions, and Statements#
In this notebook, we cover the following subjects:
Introduction to Data Types
The Print Function
Variables and Values
Operators
Expressions
Statements
1.1. Introduction to Data Types#
Python is a well-known programming language, designed by Guido van Rossum at the Centrum Wiskunde & Informatica (CWI) in Amsterdam. The design of the language allows code to be organized into reusable and self-contained objects. Knowing the three essential data types—String, Integer, and Float—is important as they are fundamental for representing and manipulating data.
A string (str) represents a sequence of characters, and is denoted using single (‘’) or double quotes (“”). A character represents any letter, digit or other symbol. Python has the following character sets:
Letters – A to Z, a to z
Digits – 0 to 9
Special Symbols - + - * / etc.
For example:
greeting = "Welcome to the first Practical ;)"
expectation = 'This will be a 10/10!'
What’s wrong with this string: tricky_string = ‘ Oops, what goes wrong here?”
?
The string starts with a single quote ’
but ends with a double quote ”
. To fix it, use matching quotes at both ends.
tricky_string = ' Oops, what goes wrong here?"
Cell In[1], line 1
tricky_string = ' Oops, what goes wrong here?"
^
SyntaxError: EOL while scanning string literal
Note
By using the #
symbol, you can add comments to your code for explanations or instructions. These lines of text are ignored during program execution.
An intiger (int) represents whole numbers.
# my first intiger
max_grade = 10
A floating-point number (float) is a number with a decimal point, for example, 3.14 or 7.0. Floats are used to represent numbers that are not whole, allowing you to work with fractions and real numbers.
# my first float
pi_number = 3.1415
What are the next two digits of pi after 3.1415?
The next two digits are 9 and 2, making it 3.1415926535…
By now, you might be asking yourself two questions:
Why does nothing show when we run our cells?
Why do we have this structure of
variable = value
?
Let’s answer the first one.
1.2. The Print Function#
The print()
function is used to display text or other information on the screen. It’s a way to show output to the user.
# What will happen when we run this cell?
print("Welcome to Intro to Python :)")
This line of code tells Python to display the text:
'Welcome to Intro to Python :)'
on the screen. You can use print()
to display numbers, text and even the results of calculations:
print(10)
print(3 + 4)
print("The sum of 3 and 4 is", 3 + 4)
Printing is handy as it allows us to visualize or display specific information. For example, by using the type()
function, we can figure out the data type of an object.
# this is an int
max_grade = 10
print(type(max_grade))
# this is an aesthetic print
print('-------------')
# this is a str
fav_animal = 'red panda'
print(type(fav_animal))
1.3. Variables and Values#
To answer the second question, we explore a fundamental concept in Python: variables. Variables in programming are often referred to as containers for data, similar to mathematical variables that store values that can later be changed. A variable in Python is created by assigning a value to a name, for which the equals sign (=
) is used. The basic syntax is:
variable_name = value
Don’t get confused on the difference between values and data types. Basically, variables store values which belong to a specific data type. For example:
# "VU" is the value of type string
university = "VU"
# "September" is the value of type string
month = "September"
# 9 is the value of type integer
month_number = 9
# 21.7 is the value of type float
degrees_celsius = 21.7
1.3.1. Type Hints#
It is good practise and mandatory in this course to add type hints to your variables. With type hints you specify the expected data type of a variable, using the syntax:
variable_name: type = value
university: str = "VU"
month: str = "September"
month_number: int = 9
degrees_celsius: float = 21.7
1.3.2. Printing with Variables#
1. f-Strings
Printed messages can include variables. A very neat way of creating print statements is f-strings, as they allow us to add the values of variables in a string. To create an f-String, you place the letter f
before the string and include the variables inside curly braces {}
. Here’s the basic syntax:
f"string {variable}"
age: int = 24000
name: str = "Gandalf"
message: str = "Welcome to Intro to Python :)"
# These variables can then be used in a print statement using an f-String:
print(f'Hi {name} ! {message} Is it difficult learning Python having the age of {age}?')
2. Format Method
Another way is to use the format
method, which allows you to set the format of the variables in your message. In the following example, we specify that we want to use three decimal places using the syntax .2f
.
expected_rain: float = 2.35
print("I'm so happy only {rain:.2f} mms of rain is expected today!".format(rain = expected_rain))
I'm so happy only 2.35 mms of rain is expected today!
1.3.3. Naming Rules of Variables#
In Python, you can’t use any arbitrary sets of characters when naming your variables; you must follow specific rules:
It should start with a letter or underscore.
It cannot start with a number.
It must only contain alpha-numeric (i.e., letters a-z A-Z and digits 0-9) characters and underscores.
They cannot share the name of a Python keyword. (Python has a set of keywords that are reserved words that cannot be used as variable names, function names, or any other identifiers. You can find them here)
What might go wrong if we uncomment and run the following code snippet?
There are three issues: variable names can’t start with a number, strings need quotes around them, and class
is a reserved keyword in Python.
# Uncomment to see what happens:
# 9th_month = "September"
# favoriteCourse = Python
# class = "tutorial"
# Legal, but not often used:
CURRENT_WIND_SPEED: int = 18
# Instead:
current_wind_speed: int = 18
EXTREME_WIND_THRESHOLD: int = 148
Note
In this course, we use snake_case as our naming convention, which means all letters are lowercase, and words are separated by underscores. Another common convention is CamelCase, where each word starts with a capital letter, and words are not separated at all.
The naming of your variables is extremely important as this improves the readability of your code. Make sure the variable name truly reflects what it represents. Below are two pieces of code that are functionally the same but differ in naming. Mind, we introduce several new concepts in this piece of code, but don’t worry about those for now. Just focus on the naming conventions of variables.
Let’s look at the proper way of naming first.
birth_year: int = 2000
current_year: int = 2024
name: str = 'Bob'
age: int = current_year-birth_year
print(f'My name is {name} and I am {age} years old')
My name is Bob and I am 24 years old
You can see how this piece of code almost reads like English, thanks to the proper naming of variables. Not convinced? Let’s check out a more abstract approach to naming things.
y: int = 2000
c: int = 2024
n: str = 'Bob'
a: int = c-y
print(f'My name is {n} and I am {a} years old')
My name is Bob and I am 24 years old
See? When the names of the variables don’t clearly reflect what they represent, it is much harder to read the code. Initially, it might be a struggle to find the right names for your variables, but you’ll get better along the way.
Note
In our notebooks we often use the variable names x
, y
, and z
in our example code. This is solely to explain a concept in a plain way and not something that is recommended in real-world applications. For your assignments, it is very likely this will even be penalized.
Which of these is a better variable name, and why? a_float = 3.1415
or pi_number = 3.1415
?
Using pi_number
is more descriptive and clearly indicates that the value is related to pi, which helps make the code easier to understand.
1.3.4. Reassignments#
It is allowed to assign a new value to an existing variable. This process is called reassignment. As soon as you assign a value to a variable, the old value is lost.
x: int = 42
print(x)
x: int = 43
print(x)
What will happen here? Correct if the output is not as expected.
university: str = "VU"
university: str = "Amsterdam"
print(university + university)
1.4. Operators#
In the last cell, we introduced something new, namely the +
operator. However, there are many more operators that can be used on variables and values.
1.4.1. Operations on Numerical Data Types#
Let’s explore the basic mathematical operators and see how they work with numerical data types.
1. Addition: +
result = 3 + 4 # Adds 3 and 4
print(result)
7
2. Subtraction: -
result = 10 - 5 # Subtracts 5 from 10
print(result)
3. Multiplication: *
result = 4 * 5 # Multiplies 4 by 5
print(result)
4. Division: /
Divides one number by another. In Python, this results in a number with a decimal point (float).
result = 20 / 4 # Divides 20 by 4
print(result)
5.0
5. Modulo: %
The Modulo operator is very useful for finding out if a number is even or odd as it computes the remainder when dividing the first integer by the second one.
result = 10 % 3 # Divides 10 by 3
print(result)
1
6. Floor division: //
Divides one number by another and rounds down to the nearest integer.
result = 20 // 3 # Divides 20 by 3 and floors the result
print(result)
7. Exponentiation: **
result = 2 ** 3 # Raises 2 to the power of 3
print(result)
1.4.2. Hierarchy of Operations#
The usual hierarchy of operations (remember the acronyms PEMDAS or BODMAS) apply for maths in Python as well. (highest on top, lowest at the bottom)
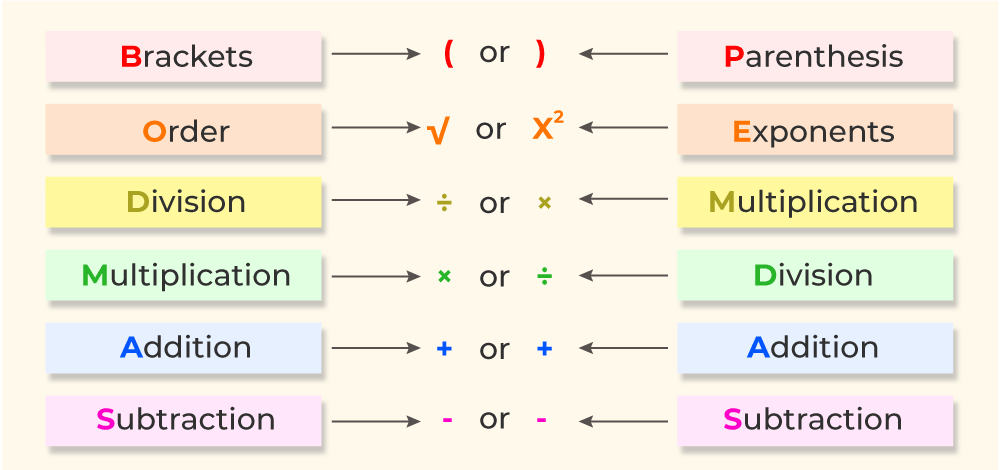
x: int = 4
y: int = 3
z: int = 2
result = x * y ** z
print(x, "*", y, "^", z, "=", result)
1.4.3. Operations on Non-Numeric Data Types#
Some operators can also be applied on non-numeric data types. For example, strings can be concatenated, so it’s perfectly fine to do this:
street: str = "De Boelelaan "
number: str = "1105"
address = street + number
print(address)
# Can you do THIS though?
address = "De Boelelaan" + 1105
print(address)
# Or THIS?
address = "De Boelelaan" - "laan" + "straat"
print(address)
# Concatenation is not the only operation that works on strings...
cheer = "Hip-" * 2
print("{cheer}Hurray, I'm done with my first Python practical!".format(cheer = cheer))
1.5. Expressions#
Now that we have covered variables, values, operators, and function calls, we can explain expressions. An expression is a combination of these elements, producing a new value. When you type an expression at the prompt, the interpreter evaluates it, which means that it calculates the value of the expression and displays it.
1. Basic Arithmetic Expression
# This expression adds the numbers 2 and 3, resulting in the value 5.
2 + 3
2. Variable Expression
# Here, x - y is an expression that subtracts y from x, resulting in 5.
x: int = 10
y: int = 5
x - y
1.6. Statements#
A statement is an instruction that has an effect, like creating a variable or displaying a value.
n: int = 17
print(n)
17
The first statement initializes the variable n
with the value 17
, this is a so-called assignment statement. The second statement is a print statement that prints the value of the variable n
.
The effect is not always visible. Assigning a value to a variable is not visible, but printing the value of a variable is.
In the following code snippet, identify the expression and the statement: circle_circumference = 2 * pi * radius
The expression is 2 * pi * radius
because it can be evaluated to produce a value. The entire snippet circle_circumference = 2 * pi * radius
is an assignment statement as it assigns the value of the expression to the variable circle_circumference
.
1.7. Exercises#
Let’s practice! Mind that each exercise is designed with multiple levels to help you progressively build your skills. Level 1 is the foundational level, designed to be straightforward so that everyone can successfully complete it. In Level 2, we step it up a notch, expecting you to use more complex concepts or combine them in new ways. Finally, in Level 3, we get closest to exam level questions, but we may use some concepts that are not covered in this notebook. However, in programming, you often encounter situations where you’re unsure how to proceed. Fortunately, you can often solve these problems by starting to work on them and figuring things out as you go. Practicing this skill is extremely helpful, so we highly recommend completing these exercises.
For each of the exercises, make sure to add type hints
, and do not import any libraries unless specified otherwise.
As we cover the most basic concepts of Python programming in this notebook, all the exercises are designed at Level 1 to help you get familiar with the fundamentals.
1.7.1. Exercise 1#
The official scientific name of a species in Latin consists of two parts: the genus name and the species epithet. However, in English, we often refer to animals by their common names.
Level 1: Write a program that, given the genus, species epithet, and English name of an animal, prints both the full Latin name and the English name of the animal. Do not use the .format()
method!
Example input:
genus: str = 'Felis'
epithet: str = 'catus'
english_name: str = 'cat'
Example output:
'Felis catus is a scientific name for a cat.'
# TODO.
1.7.2. Exercise 2#
Level 1: Let’s create a program that prints the message ‘Happy birthday’ followed by the person’s name. The message should be repeated as many times as the age they are turning.
Example input:
name: str = 'Mia'
age: int = 6
Example output:
'Happy birthday Mia! Happy birthday Mia! Happy birthday Mia! Happy birthday Mia! Happy birthday Mia! Happy birthday Mia!'
# TODO.
1.7.3. Exercise 3#
Assume you are a cashier who needs to calculate the amount of money to return to a customer as change. However, you have a special cash drawer that only contains dollars, quarters, and dimes.
Level 1: Write a program that uses the modulo operator to calculate the number of dollars, quarters, and dimes to be given when a customer needs to receive €8.60 in change.
The output should be a string in the following format:
'Cashier has to give back: 8 dollar(s), 2 quarter(s), and 1 dime(s).'
Hints:
1 nickel is worth 5 pennies
10 pennies equals 1 dime
25 pennies equals 1 quarter
100 pennies equals 1 dollar
# TODO.
Material for the VU Amsterdam course “Introduction to Python Programming” for BSc Artificial Intelligence students. These notebooks are created using the following sources:
Learning Python by Doing: This book, developed by teachers of TU/e Eindhoven and VU Amsterdam, is the main source for the course materials. Code snippets or text explanations from the book may be used in the notebooks, sometimes with slight adjustments.